Overview
Introduction
This tutorial walks through the process of creating a Discord webhook and triggering it with a custom Python script. It expects that you already have a server with admin privileges. If you don't, you can create your own free Discord server at https://discordapp.com.
Create the Webhook
First you have to create webhook. You create the webhook from within the Discord app. Open the server settings for a server that you manage.
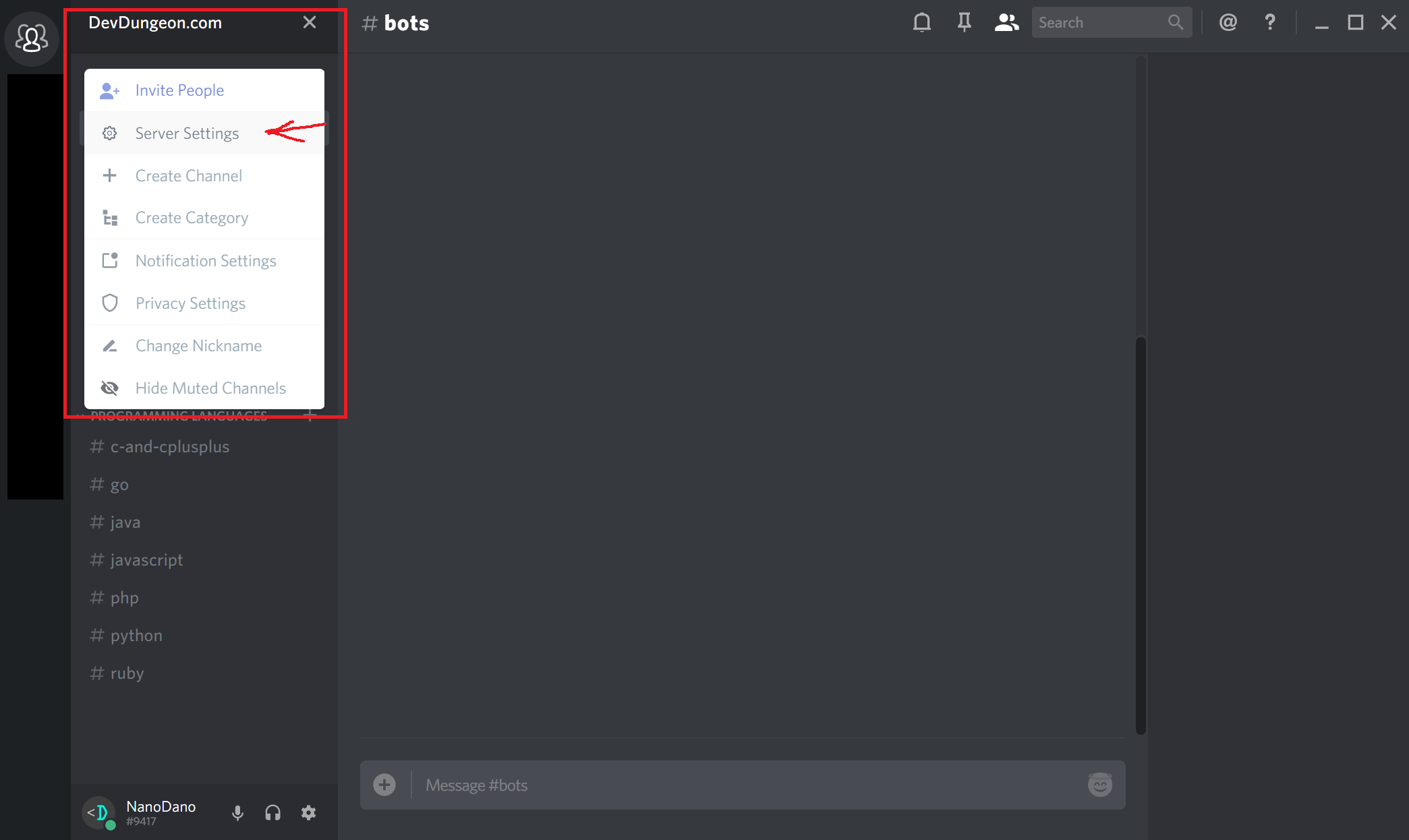
In the server settings menu, go to the webhooks tab and create a new webhook.
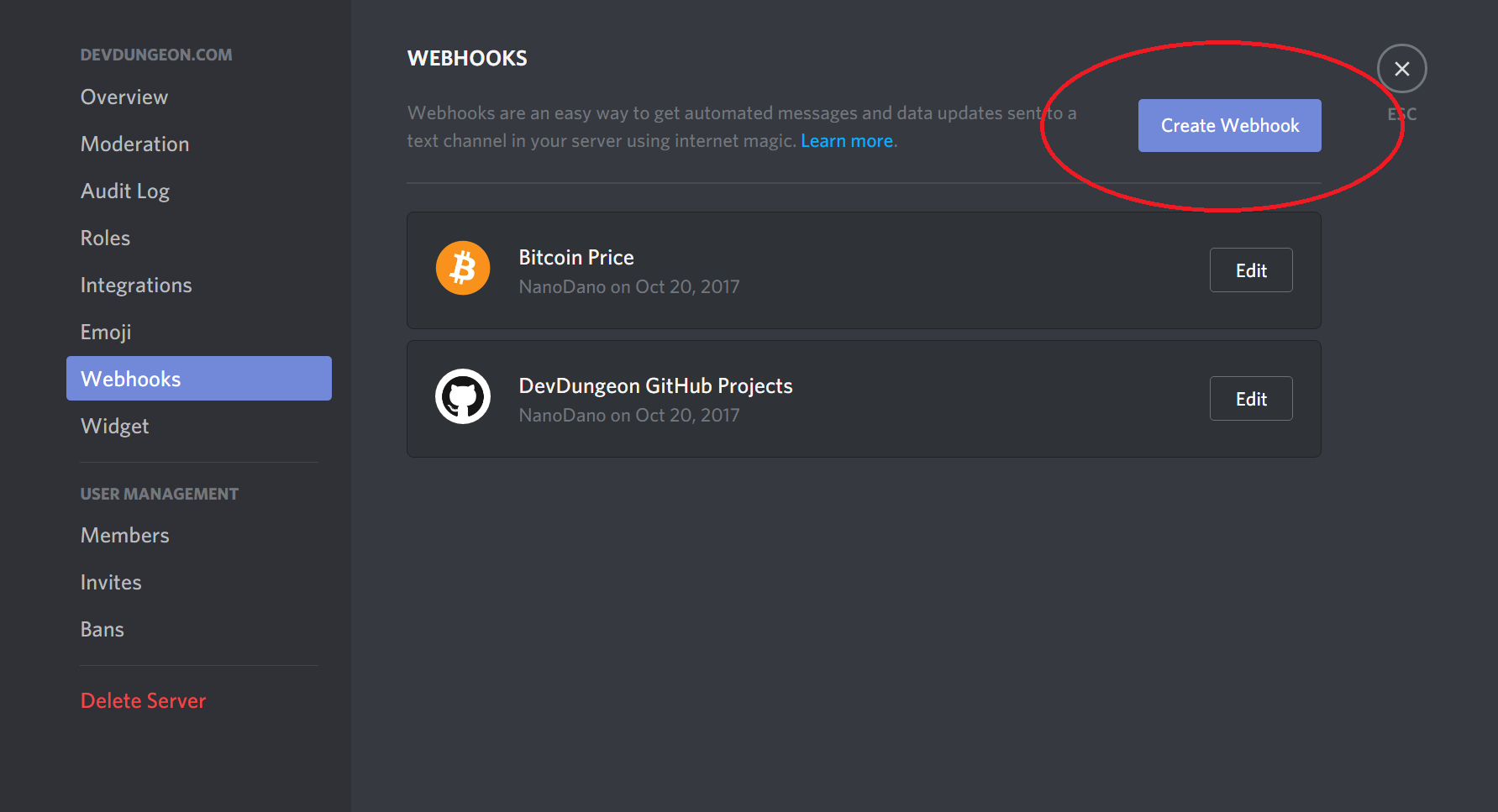
On the "Create Webhook" menu, configure the options as desired. Give it a name, upload an avatar, and choose the channel you want it to post to. Copy the webhook URL as you will need it later.
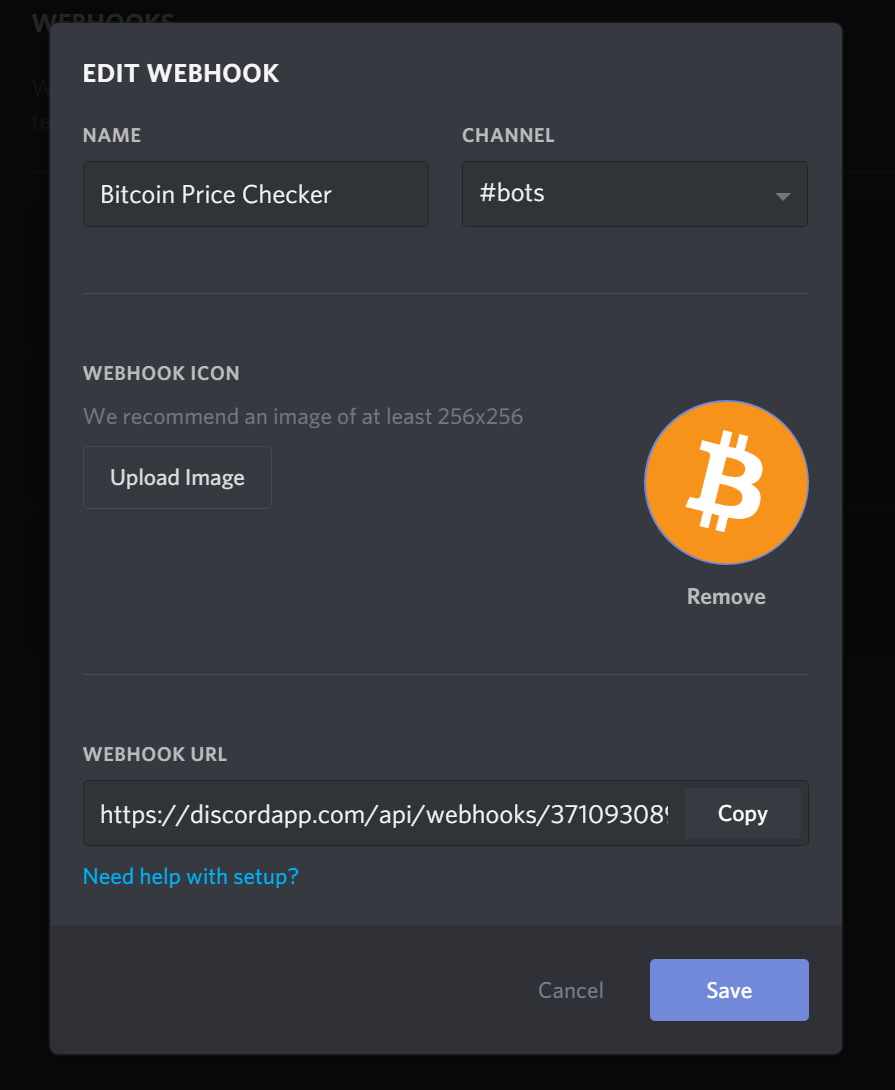
Create the Python Script
Now create a new Python file.
# discord_bitcoin_price_bot.py
import requests
# Provide the webhook URL that Discord generated
discord_webhook_url = 'https://discordapp.com/api/webhooks/<webhookid>/<tokenid>'
# Get the BTC price from CoinDesk
bitcoin_price_url = 'https://api.coindesk.com/v1/bpi/currentprice/BTC.json'
data = requests.get(bitcoin_price_url).json()
price_in_usd = data['bpi']['USD']['rate']
# Post the message to the Discord webhook
data = {
"content": "Bitcoin price is currently at $" + price_in_usd + " USD"
}
requests.post(discord_webhook_url, data=data)
Execute the Script
python discord_bitcoin_price_bot.py
Conclusion
That is it! All you have to do is run the script and it will make an HTTP POST request to your webhook URL which will post a message to Discord. Whenever you run this Python script it will post the current Bitcoin price to the Discord channel. If you want to run this script automatically at certain time intervals, consider setting it up as a cron job.
Also check out the other options available at Discord "Execute Webhook" Reference. You have the option to override the username and avatar image as well as upload a file attachment.